Sometimes we need to display a messagebox to user. Especially when user interaction is required and we want to warn user or inform that a process has completed or so. Powershell leverages all .NET features available to any .NET language such as C# or VB.NET. This gives a lot of freedom to developers and there are not console world and GUI world when you are programming with Powershell. Let's take a look how we can do it.
Below video is the complete demonstation:
Generating a Messagebox -
1. Load the Assembly
[System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
Output-
GAC Version Location
--- ------- --------
True v2.0.50727 C:\Windows\assembly\GAC_MSIL\System.Windows.Forms\2.0.0.0__b77a5c561934e089\System.Windows.Forms.dll
Note: If you don't want the output, you can simple redirect to Out-nul. This will skip displaying assembly loading statement.
You can also cast is with [void] such as below:
[void][System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
2. Display a simple Messagebox
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step.")
Now, the messagebox appears something like this -
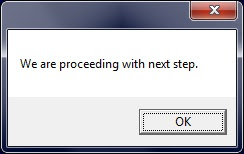
If you see above message, you will find Title is missing. Let's add a title also by adding below piece of code -
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status")
So, this was all about showing message with title. This was just OK message so, there is nothing to decide for user except pressing OK button.
Types of Messageboxes :
We have 6 types of Messageboxes in Powershell -
Note: The number mentioned in left is the third parameter of Messagebox.
If you want to show Yes No, just add 4 as third parameter -
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status" , 4)
Now, this will display a Messagebox like this -
How to get values from Messagebox?
As you know, when you press any button, you need to get the result and work upon the decision -
$OUTPUT= [System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status" , 4)
if ($OUTPUT -eq "YES" )
{
..do something
}
else
{
..do something else
}
The value of button pressed is stored in $OUTPUT variable. This variable can then be used for your programming logic.
I have given just a primer how to use Messagebox class. But, if you want to go indepth of System.Windows.Forms.MessageBox class, you may look for the link below -
http://msdn.microsoft.com/en-us/library/system.windows.forms.messageboxbuttons.aspx
Below video is the complete demonstation:
Generating a Messagebox -
1. Load the Assembly
[System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
Output-
GAC Version Location
--- ------- --------
True v2.0.50727 C:\Windows\assembly\GAC_MSIL\System.Windows.Forms\2.0.0.0__b77a5c561934e089\System.Windows.Forms.dll
Note: If you don't want the output, you can simple redirect to Out-nul. This will skip displaying assembly loading statement.
You can also cast is with [void] such as below:
[void][System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
2. Display a simple Messagebox
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step.")
Now, the messagebox appears something like this -
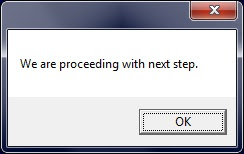
If you see above message, you will find Title is missing. Let's add a title also by adding below piece of code -
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status")
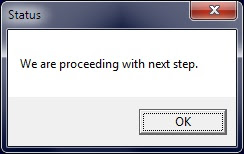
So, this was all about showing message with title. This was just OK message so, there is nothing to decide for user except pressing OK button.
Types of Messageboxes :
We have 6 types of Messageboxes in Powershell -
0: | OK |
1: | OK Cancel |
2: | Abort Retry Ignore |
3: | Yes No Cancel |
4: | Yes No |
5: | Retry Cancel |
Note: The number mentioned in left is the third parameter of Messagebox.
If you want to show Yes No, just add 4 as third parameter -
[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status" , 4)
Now, this will display a Messagebox like this -
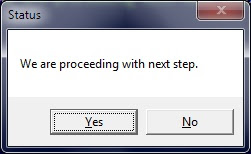
How to get values from Messagebox?
As you know, when you press any button, you need to get the result and work upon the decision -
$OUTPUT= [System.Windows.Forms.MessageBox]::Show("We are proceeding with next step." , "Status" , 4)
if ($OUTPUT -eq "YES" )
{
..do something
}
else
{
..do something else
}
The value of button pressed is stored in $OUTPUT variable. This variable can then be used for your programming logic.
I have given just a primer how to use Messagebox class. But, if you want to go indepth of System.Windows.Forms.MessageBox class, you may look for the link below -
http://msdn.microsoft.com/en-us/library/system.windows.forms.messageboxbuttons.aspx
Nice Work! Exactly what I was happening to be looking for this afternoon. Great Job!
ReplyDeleteThanks for the comment!
DeleteI like this a lot, thanks for publishing. Can you help me with part of your script. The ...do somethine else. I want it to call a .bat file in a different location. can you show me how to do that please.
ReplyDeleteHi John,
ReplyDeleteTo call a bat file from Powershell, you may use below lines (Assuming that batch file is named 'som.bat'. Below line will be sufficient:
&cmd /c som.bat
Description:
& is for calling any executable inside Powershell.
/c is to run the statement and terminate command shell. Eventually it will return to Powershell.
Thank you for publishing this post.
ReplyDeleteAlex D.
I just used this to make a messagebox appear when there's an error in a powershell script that emails me the results... Very useful! I don't need to go and check the results, as the messagebox tells me! Very useful :)
ReplyDeleteI have an issue in which ISE wants to hide the MessageBox. I have tried a variety of different methods, but must be missing something. Here is my code snippet.
ReplyDeleteFunction Show-MessageBoxA
{
[System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms") | Out-Null
$responseA=[System.Windows.Forms.MessageBox]::Show("You Have Selected Logfile - $fileName - Is This Correct?", "Status", 4)
Set-Variable -Name _ResponseA ($responseA) -Scope "Global"
}
Thanks
known issue still happening in 2016
DeleteHappens here too...
DeleteThank's for useful and well-written blog! If I may add, you can also use the "wshell"
ReplyDeleteSample code based on http://gallery.technet.microsoft.com/scriptcenter/1a386b01-b1b8-4ac2-926c-a4986ac94fed ( at least for PowerShell Versions >= 3.0 )
$a = new-object -comobject wscript.shell
$intAnswer = $a.popup("Hello World!", `
0,"Info",0)
Best Regards
Eirik
great tutorial, simple and concise.
ReplyDeletecheers
Damian
Thanks you for this information about message box, glad to visit this post.
ReplyDeleteDisplay Boxes
Very simple and useful. Thanks for sharing!
ReplyDeleteVery helpful, Thanks
ReplyDeleteGood post, thank you.
ReplyDeletei want to see the current time in a messagebox. i have sheduled the script. it is also displaying the powershell prompt window along with the message box. how to hide it?
ReplyDeleteThanks in advance for valuable suggestions
I tried this on a Windows 10 Professional desktop and I did not have to load the assembly first. I just called [System.Windows.Forms.MessageBox]::Show("We are proceeding with next step.")
ReplyDeletehow to create a message box with 2 column. i want 1-50 in 1 col and 50 to 100 in another.
ReplyDeleteGreat post.
ReplyDeleteGreat, clear explanation. Just what i was looking for. Thanks!!
ReplyDeleteGreat post.
ReplyDeleteHi, I am using same code,
ReplyDeleteNow I want massagebox to be closed automatically after specified time, please help me for the same.
Regards
Pankaj
well can some one help me with the script for displaying the following message when one plugins the usb /storage device in the system " "Warning, data stored on USB drives is prone to viruses and can be easily lost. Did you know you have unlimited storage on Google Drive?"
ReplyDeleteAs i want to do it on 600 + PCS it will be great help if it can be pushed through the Active directory .
Thanks
Parvez Kamliwale
Nice blog. Thanks for sharing useful infomation. Pop-up Display System
ReplyDeleteThe possible answers to a MessageBox are one of these:
ReplyDelete[system.enum]::getNames([System.Windows.Forms.MessageBoxButtons])
Been looking all over the internet, and this is what I was looking for. Simple and easy and i've now been able to finish my code.
ReplyDeleteThanks :)
ReplyDeleteMy name is Leah Brown, I'm a happy woman today? I told myself that any loan lender that could change my life and that of my family after having been scammed separately by these online loan lenders, I will refer to anyone who is looking for loan for them. It gave me and my family happiness, although at first I had a hard time trusting him because of my experiences with past loan lenders, I needed a loan of $300,000.00 to start my life everywhere as single mother with 2 children, I met this honest and God fearing online loan lender Gain Credit Loan who helped me with a $300,000.00 loan, working with a loan company Good reputation. If you are in need of a loan and you are 100% sure of paying the loan please contact (gaincreditloan1@gmail.com)
How can I actually make the system reboot in 2 minutes after ok was clicked in the dialog box buttons?
ReplyDeleteUse enum string values instead of magic constants
ReplyDelete[System.Windows.Forms.MessageBox]::Show("We are proceeding with next step.", "Status", "OK", "Exclamation")
The possible enumeration values are "OK,OKCancel,AbortRetryIgnore,YesNoCancel,YesNo,RetryCancel".
Deleteand "None,Hand,Error,Stop,Question,Exclamation,Warning,Asterisk,Information"
amazing site thanks for sharing with us
ReplyDeletevisit our website for customize boxes
great site
ReplyDeletevisit our website for boxes
This comment has been removed by a blog administrator.
ReplyDelete